Sunday, December 24, 2017
Codes for file upload in PHP (Step 3)
In this example, we will upload file to the designated location. So, we will create a folder to store the files we've uploaded and give name to that folder. I give it as tnw87. Ok, lets start write the following codes and discover how it works.
<?php
$name = $_FILES['file']['name'];
$type = $_FILES['file']['type'];
$size = $_FILES['file']['size'];
$tmp_name = $_FILES['file']['tmp_name'];
if(@isset($name) && @!empty($name)){
$location = 'tnw87/';
if(move_uploaded_file($tmp_name, $location.$name)){
echo $name. ' is successfully uploaded.';
}else{
echo 'Upload Errors';
}
}else{
echo 'Please select file to upload';
}
?>
Wednesday, December 20, 2017
Codes for file upload in PHP (Step 2)
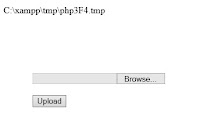
When we upload a file into one location, the file is firstly saved in temporary location as a temp file. Ok, lets discover how it works. We will write the following codes and test it.
<?php
$name = $_FILES['file']['name'];
$type = $_FILES['file']['type'];
$size = $_FILES['file']['size'];
$tmp_name = $_FILES['file']['tmp_name'];
if(@isset($name) && @!empty($name)){
$location = 'tnw87/';
if(move_uploaded_file($tmp_name, $location.$name)){
echo $name. ' is successfully uploaded.';
}else{
echo 'Upload Errors';
}
}else{
echo 'Please select file to upload';
}
?>
<style type = "text/css">
form{margin-top : 100px; margin-left : 50px;}
</style>
<form action = "upload.php" method = "POST" enctype = "multipart/form-data">
<input type = "file" name = "file"></br></br>
<input type = "submit" value = "Upload">
</form>
Friday, December 15, 2017
Codes for File Upload in PHP (Step 1)
This is the first step to write codes for uploading file in php. In this example, you can check name, type and size of files you upload.
<?php
@$name = $_FILES['file']['name'];
@$type = $_FILES['file']['type'];
@$size = $_FILES['file']['size'];
if(@isset($name) && @!empty($name)){
echo 'File name is <strong> '.$name.'</strong>, File type is <strong>'.$type.'</strong> nad File size is <strong> '.$size.'</strong>';
}else{
echo 'Please select file to upload';
}
?>
<?php
@$name = $_FILES['file']['name'];
@$type = $_FILES['file']['type'];
@$size = $_FILES['file']['size'];
if(@isset($name) && @!empty($name)){
echo 'File name is <strong> '.$name.'</strong>, File type is <strong>'.$type.'</strong> nad File size is <strong> '.$size.'</strong>';
}else{
echo 'Please select file to upload';
}
?>
Thursday, December 14, 2017
Configuring Frame Relay in Cisco Devices (Example 2)
#Router 1#
enconfig t
hostname R1
int g0/0
ip address 192.168.1.1 255.255.255.0
no shut
int s0/0/0
description Link From Router1 to Router2
ip address 223.128.1.1 255.255.255.252
encap frame-relay
frame-relay lmi-type cisco
frame-relay interface-dlci 101
no shut
int s0/0/1
encap frame-relay
no shut
exit
int s0/0/1.201 point-to-point
description Link From R1 to R3
ip address 223.128.1.5 255.255.255.252
frame-relay interface-dlci 201
no shut
exit
int s0/0/1.301 point-to-point
description Link From R1 to R4
ip address 223.128.1.9 255.255.255.252
frame-relay interface-dlci 301
no shut
exit
int s0/0/1.401 point-to-point
description Link From R1 to R5
ip address 223.128.1.13 255.255.255.252
frame-relay interface-dlci 401
no shut
exit
int s0/0/1.501 point-to-point
description Link From R1 to R6
ip address 223.128.1.17 255.255.255.252
frame-relay interface-dlci 501
no shut
exit
router rip
version 2
network 192.168.1.0
network 223.128.1.0
network 223.128.1.4
network 223.128.1.8
network 223.128.1.12
network 223.128.1.16
end
copy run start
Wednesday, December 13, 2017
Configuring Frame Relay in Cisco Devices (Example 1)
***Router1***
enconfig t
hostname Router1
int s0/0/0
encap frame-relay
no shut
exit
int s0/0/0.102 point-to-point
description Link From Router1 to Router2
ip address 223.200.100.5 255.255.255.252
frame-relay interface-dlci 102
no shut
exit
int s0/0/0.103 point-to-point
description Link From Router1 to Router3
ip address 223.200.100.9 255.255.255.252
frame-relay interface-dlci 103
no shut
exit
router rip
version 2
network 223.200.100.4
network 223.200.100.8
end
copy run start
Tuesday, December 12, 2017
Using XML with PHP (Example 2)
In this example, you will see using foreach function twice. Ok, let's start write codes. We will write the following xml codes and save it as file name 'example.xml'.
<producer>
<producer>
<food>
<foodname>banana</foodname>
<foodprice>100 kyats</foodprice>
</food>
</producer>
<producer>
<food>
<foodname>lime</foodname>
<foodprice>200 kyats</foodprice>
</food>
</producer>
<producer>
<food>
<foodname>milk</foodname>
<foodprice>300 kyats</foodprice>
</food>
</producer>
</producer>
<producer>
<producer>
<food>
<foodname>banana</foodname>
<foodprice>100 kyats</foodprice>
</food>
</producer>
<producer>
<food>
<foodname>lime</foodname>
<foodprice>200 kyats</foodprice>
</food>
</producer>
<producer>
<food>
<foodname>milk</foodname>
<foodprice>300 kyats</foodprice>
</food>
</producer>
</producer>
Monday, December 11, 2017
Using XML with PHP (Example 1)
We will write the following codes and save it as file name 'example.xml'.
<producer>
<producer>
<name>Thet</name>
<age>30</age>
</producer>
<producer>
<name>Jan</name>
<age>21</age>
</producer>
<producer>
<name>Feb</name>
<age>23</age>
</producer>
<producer>
<name>Mar</name>
<age>25</age>
</producer>
</producer>
<producer>
<producer>
<name>Thet</name>
<age>30</age>
</producer>
<producer>
<name>Jan</name>
<age>21</age>
</producer>
<producer>
<name>Feb</name>
<age>23</age>
</producer>
<producer>
<name>Mar</name>
<age>25</age>
</producer>
</producer>
Friday, December 8, 2017
WordCensoring in PHP
From the following example, we can learn the usage of form, textarea, isset, strtolower and str_replace. Every word 'love' we type in the text box, the codes will change with the word 'hate'.
<?php
$find = 'love';
$replace = 'hate';
if(isset($_POST['user_input']) && !empty($_POST['user_input'])){
$user_input = strtolower($_POST['user_input']);
$user_input_new = str_replace($find, $replace, $user_input);
echo $user_input_new.'</br>';
}else{
echo 'Please fill in the text box!';
}
?>
<?php
$find = 'love';
$replace = 'hate';
if(isset($_POST['user_input']) && !empty($_POST['user_input'])){
$user_input = strtolower($_POST['user_input']);
$user_input_new = str_replace($find, $replace, $user_input);
echo $user_input_new.'</br>';
}else{
echo 'Please fill in the text box!';
}
?>
Thursday, December 7, 2017
Configuring OSPF in Cisco Routers
*** Router 1 ***
Activate the connection to PC1
enconfig t
int g0/0
ip address 192.168.1.1 255.255.255.0
no shut
Activate the connection to Router2
int s0/0/1
ip address 223.200.100.9 255.255.255.252
clock rate 64000
no shut
Activate the connection to Router 3
int s0/0/0
ip address 223.200.100.1 255.255.255.252
no shut
exit
Configure OSPF
router ospf 10
network 192.168.1.0 0.0.0.255 area 0
network 223.200.100.0 0.0.0.3 area 0
network 223.200.100.8 0.0.0.3 area 0
end
Save the configuration
copy run start
Wednesday, December 6, 2017
String Trim in PHP
<?php
$string = 'I am Thet Naing Win.';
$string_trimmed = rtrim($string);
echo $string_trimmed;
?>
$string = 'I am Thet Naing Win.';
$string_trimmed = rtrim($string);
echo $string_trimmed;
?>
Tuesday, December 5, 2017
Configuring PPP in Cisco Routers
This is the configuration of PPP (PAP) in Cisco Routers
*** Mandalay Router ***
enconfig t
username Mandalay password Mandalay
hostname Mandalay
int s0/0/0
ip address 223.200.100.1 255.255.255.252
no shut
encap ppp
ppp authentication pap
ppp pap sent-username Naypyidaw password Naypyidaw
exit
int s0/0/1
ip address 223.200.100.9 255.255.255.252
no shut
encap ppp
ppp authentication pap
ppp pap sent-username Yangon password Yangon
exit
router rip
version 2
network 223.200.100.0
end
copy run start
Monday, December 4, 2017
String Word Count in PHP
<?php
$string = 'I am Thet Naing Win and who are you?';
$string_word_count = str_word_count($string, 1);
print_r($string_word_count);
?>
$string = 'I am Thet Naing Win and who are you?';
$string_word_count = str_word_count($string, 1);
print_r($string_word_count);
?>
Sunday, December 3, 2017
Writing Basic XML Codes
*** Example 1 ***
<producer>
<producer>
<name>Thet</name>
<age>30</age>
</producer>
<producer>
<name>Naing</name>
<age>32</age>
</producer>
<producer>
<name>Win</name>
<age>33</age>
</producer>
</producer>
<producer>
<producer>
<name>Thet</name>
<age>30</age>
</producer>
<producer>
<name>Naing</name>
<age>32</age>
</producer>
<producer>
<name>Win</name>
<age>33</age>
</producer>
</producer>
Saturday, December 2, 2017
Virtualization (Installing Ubuntu in VirtualBox)
Virtualization
Virtualizatin is installing two or more operating systems in one physical machine. The main effects of using virtualizaton are security and cost saving. Oracle VirtualBox is one of virtualization softwares. It is open source software and totally free. Please click here to download. Install it after downloading.Ubuntu
Ubuntu is one of open source operating systems. Please click here to download ubuntu ISO file.Installing Ubuntu in VirtualBox
1. Start VirtualBox
- Open VirtualBox and Click New.
2.Choose Name and Operating System
- Type name in the Name box (for example, T N W)
- Choose operating system in Type box (here we will choose Linux)
- Chose version in Version box (here I will choose Ubuntu-64 bit)
- And then click Next.
Friday, December 1, 2017
String Replace in PHP
<?php
$string = 'I am thet naing win';
$find = array('thet', 'naing', 'win');
$replace = array('THET', 'NAING', 'WIN');
$string_new = str_replace($find, $replace, $string);
echo $string_new;
?>
Wednesday, November 29, 2017
Disable Auto Update in Windows 10
Some of us may face the problems that the windows is getting updated while we are doing urgent jobs. Or the windows is getting updated while we prepare to go back home at the time when the office is closed and the power is going to be shut down. To stop that kind of problem, we should disable auto update in windows.
To disable auto update in windows by command line
- Run command prompt as administrator
- Type net stop wuauserv in the command prompt and press enter
- Type net stop bits and press enter
- Type net stop dosvc and press enter
Sunday, November 26, 2017
Header in PHP
<?php
$redirect_page = 'http://www.tnw87.com';
$redirect = true;
if($redirect == true)
{
header('location:' .$redirect_page');
}
?>
<h1>Your connection is wrong in something</h1>
$redirect_page = 'http://www.tnw87.com';
$redirect = true;
if($redirect == true)
{
header('location:' .$redirect_page');
}
?>
<h1>Your connection is wrong in something</h1>
Timestamp in PHP
<?php
$time = time();
$time_current = date('d M Y @ H:i:s', $time);
$time_modified = date('d M Y @ H:i:s', tostrtime(+ 1 year));
echo 'The current time is ' .$time_current. ' and The time modified is ' .$time_modified.'!';
?>
$time = time();
$time_current = date('d M Y @ H:i:s', $time);
$time_modified = date('d M Y @ H:i:s', tostrtime(+ 1 year));
echo 'The current time is ' .$time_current. ' and The time modified is ' .$time_modified.'!';
?>
Thursday, November 23, 2017
String Lower / Upper in PHP
We can change all characters to lower case by using strtolower. The following code is an example.
<?php
$string = 'I am ThEt NainG WiN!';
$string_low = strtolower($string);
echo $string_low;
?>
<?php
$string = 'I am ThEt NainG WiN!';
$string_low = strtolower($string);
echo $string_low;
?>
Wednesday, November 22, 2017
Securing Cisco Networking Devices
We should set passwords in Cisco networking devices so that non authorized people can't access easily. The followings are the ways to set passwords in Cisco networking devices by CLI.
Console Password
enable (en)
configure terminal (config t)
line console 0
password console123 (type secured password)
Tuesday, November 21, 2017
Checking DNS (Domain Name System)
We can use nslookup command to check dns (domain name system). How to check:
In windows,
- Press Windows + R keys together.
- Type cmd in the run box and press enter.
- Type nslookup in the command prompt and press enter.
To check dns:
- Type example.com and press enter. If your dns is working, you will see Name and IP Address.
To check website:
- Type www.example.com and press enter. If your website is working, you will see your hosting name and IP Address.
In windows,
- Press Windows + R keys together.
- Type cmd in the run box and press enter.
- Type nslookup in the command prompt and press enter.
To check dns:
- Type example.com and press enter. If your dns is working, you will see Name and IP Address.
To check website:
- Type www.example.com and press enter. If your website is working, you will see your hosting name and IP Address.
Do While Loop in PHP
<?php
$count = 1;
$message = 'Hello!';
do {
echo $count.'. '.$message.'</br>';
$count ++;
}
while($count<=10)
?>
Friday, November 17, 2017
While Loop in PHP
<?php
$count = 1;
$message = 'I Love You!';
while($count<=10){
echo $count. '.' .$message.'</br>';
$count ++;
}
?>
Thursday, November 16, 2017
If Statement in PHP
<?php
$num1 = 30;
$num2 = 40;
if($num1 > $num2){
echo 'Num1 is greater than Num2!';
} else if($num1 == $num2){
echo 'Num1 and Num2 are equal!';
}else{
echo 'Num2 is greater than Num1!';
}
?>
Wednesday, October 11, 2017
String Length in PHP
<?php
$string = 'I am Thet Naing Win';
$strlen = strlen($string);
echo $strlen;
?>
Tuesday, October 10, 2017
String Position in PHP
<?php
$string = 'I am Thet Naing Win. This is PHP Testing and Word position Test. If you are insterested in PHP, please join us!';
$find = 'T';
$find_length = strlen($find);
$offset = 1;
while ($strpos = strpos($string, $find, $offset)){
echo 'The Character <strong>'.$find. '</strong> is found at '.$strpos.'.</br>';
$offset = $strpos + $find_length;
}
?>
Thursday, October 5, 2017
HTML Form
?>
<head>
<title>html form</title>
<style type="text/css">
form{border:dotted; width:300px; height:auto; color: orange; text-align: center; margin-top:300px;}
</style>
</head>
<form action="index.html" method="POST">
<br />
UserName<br />
<input type="text" name="username"><br /><br />
Password<br />
<input type="password" name="password"><br /><br />
<input type="submit" value="Log In">
<br /><br />
</form>
Saturday, April 29, 2017
Knowledge About Hard Disk
There are various types of Hard Disks. Some of them are:
IDE : Integrated Device Electronic. Known as PATA as well.
PATA : Parallel advance technology attachment
- Mostly found in Desktop computers. But, don't use so much nowadays.
SATA: Serial advance technology attachment
- Used in Laptops, Desktops and Servers.
SCSI: Small Computer System Interface
SAS: Serial attached SCSI
- Mostly found in Servers.
Thursday, April 27, 2017
Using Computer Management Service in Windows
How to Open Computer Management Service Windows
- Press Windows and R keys together at the same time.
- Type compmgmt.msc in the run box and click OK.
Then, Computer Management Windows will be appeared.
There are Task Scheduler, Event Viewer, Shared Folders, Performance, Device Manager, Disk Management and Internet Information Services (IIS) Manager.
Task Scheduler
We can create and manage tasks in our computer.
Using Component Services In Windows
How to open component services in Windows
- Press Windows and R keys together at the same time.
- Type comexp.msc in the run box and click OK.
Then, component services window will be appeared.
In component services, we can use Event Viewer, Windows Logs and Services.
In Event Viewer, we can check all the events happened in our computers.
In Windows Logs, we can check the logs of Application, Security, Setup, System and Forward Event.
Wednesday, April 26, 2017
How to Configure EIGRP in Cisco Router
Router 1
Activate the connection to PC1
en
config t
int g0/0
ip address 192.168.1.1 255.255.255.0
no shut
Activate the connection to Router2
int s0/0/1
ip address 223.200.100.9 255.255.255.252
clock rate 64000
no shut
Activate the connection to Router 3
int s0/0/0
ip address 223.200.100.1 255.255.255.252
no shut
exit
Configure EIGRP
router eigrp 10
network 192.168.1.0 0.0.0255
network 223.200.100.0 0.0.0.3
network 223.200.100.8 0.0.0.3
end
Save the configuration
copy run start
Friday, April 14, 2017
How to configure RIP in Cisco Routers
RIP (Routing Information Protocol) is the oldest distance vector routing protocol. The maximum number of hop count is 15 and the hop count number 16 is defined as infinite distance. The converged time is slow if compared with other routing protocols such as EIGRP, OSPF and IS-IS. There are two versions in RIP: version 1 and version 2.
RIP V1
RIP v1 uses FLSM (fixed length subnet masking) and does not support VLSM(variable length subnet masking). Using FLSM causes IP wastes.
FLSM Examples,
192.168.1.0 /24
192.168.2.0 /24
RIP V2
RIP V2 supports VLSM (variable length subnet masking). If there are different amount of hosts in an organization, we should use RIP v2.
For example,
HR Department : 20 hosts
Engineering Department: 50 hosts
IT department: 10 hosts
Sales department: 30 hosts
The following is the sample configuration of RIP in Cisco Routers.
Wednesday, April 12, 2017
DHCP Configuration in Cisco Router
DHCP is dynamic host configuration protocol. By configuration DHCP in a router, we don't need to give IPs to the hosts manually. DHCP automatically defines IPs for hosts. We can also configure DHCP in Cisco Routers as well.
The following is the sample configuration of DHCP in Cisco Router:
* Router *
en
config t
int g0/0
ip address 192.168.1.1 255.255.255.0
no shut
exit
ip dhcp pool Test
network 192.168.1.0 255.255.255.0
default-router 192.168.1.1
dns-server 192.168.1.1
end
copy run start
If you want to see sample configuration in packet tracer, you can download pkt file. Click here.
(Note: you need to have packet tracer installed in your computer to open pkt file).
Monday, April 10, 2017
String Reverse In PHP
<?php
$string = 'I am Thet Naing Win';
$string_reversed = strrev($string);
echo $string_reversed;
?>
Sunday, April 9, 2017
Cisco EtherChannel
EtherChannel is combination of two or more physical ports into one logical/virtual port for redundancy or bandwidth needs.
Click here to download pkt file.
Note: You need cisco packet tracer to open pkt file.
How To Configure EtherCahnnel in Cisco Switches:
Switch1
Step 1 : Give Name to Switch 1
en
config t
hostname IT
Step 2 : Create VLan and give name to it
vlan 20
name IT
exit
Step 3 : Create Etherchannel
interface range f0/1 - 4
switchport mode access
switchport access vlan 20
channel-group 5 mode auto
end
Thursday, April 6, 2017
Dell 5420
Some of my friends ask me about fair priced good used laptops. So, I post this post. This laptop is not bad for those who are looking for good used laptops with faired price. This laptop is good enough for office use.
Randomization in PHP
<?php
if(isset($_POST['roll'])){
$rand = rand(000, 999);
echo 'You roll a '.$rand;
}
?>
<form action="rand.php" method="POST">
<input type="submit" name="roll" value="Roll a Dice">
</form>
Ob Start in PHP
<?php ob_start(); ?>
<h1>Test</h1>
This is PHP Testing!
<?php
$direct_page = 'http://www.tnw87.com';
$direct = true;
if($direct == true){
header('location: '.$direct_page);
}
ob_start_flush();
?>
Sunday, April 2, 2017
Matching With Function In PHP
<?php
$string = 'I am Thet Naing Win and who are you?';
$find = '/000/';
function Match($string){
global $string;
global $find;
if(preg_match($find, $string)){
return true;
}else{
return false;
}
}
if(Match($string)){
echo 'Match Found!';
}else{
echo 'Match Not Found!';
}
?>
Matching In PHP
<?php
$string = 'Hi How are you?. I am Thet Naing Win. Nice to meet you!';
$find = '/000/';
if (preg_match($find, $string)){
echo 'Match Found!';
}else{
echo 'Match Not Found!';
}
?>
How To Use foreach In PHP
$food = array('Healthy'=>array('Salad', 'Milk', 'Coconet', 'Ground_nut'),
'Unhealthy'=>array('Alcohol', 'Beer', 'Ice_cream'));
foreach($food as $element => $inner_element){
echo '</br><strong>'.$element.'</strong></br>';
foreach($inner_element as $item){
echo $item.'</br>';
}
}
?>
Saturday, April 1, 2017
Array In PHP
<?php
$snack = array('Banana', 'Ice-cream', 'Coconet', 'Mango', 'Orange');
$snack[1]='Alcohol';
echo $snack[1];
//print_r($snack);
?>
Function In PHP
<?php
function Add($num1, $num2){
$result = $num1 + $num2;
return $result;
}
function Divide($num1, $num2){
$result = $num1 / $num2;
return $result;
}
$sum = Add(Add(10, 10), Divide(14, 2));
echo $sum;
?>
Saturday, March 25, 2017
Calculating IP Address (Version 4)
There are four octet in IPV4. Each octet has 8 bits. So, there are 32 bits in IPV4. IPV4 is based on binary (0, 1) and decimal (0 to 9).
1st Octet 2nd Octet 3rd Octet 4th Octet
8 bits 8 bit 8 bits 8 bits
27 26 25 24 23 22 21 20 27 26 25 24 23 22 21 20 27 26 25 24 23 22 21 20 27 26 25 24 23 22 21 20
255.255.255.255 255.255.255.255 255.255.255.255 255.255.255.255
How become 255.255.255.255
27 = 128 , 26 = 64 , 25 = 32 , 24 = 16 , 23 = 8 , 22 = 4 , 21 = 2 , 20 = 1
128 + 64 + 32 + 16 + 8 + 4 + 2 + 1= 255
Decimal Binary
192.168.1.0 = 11000000 . 10101000 . 00000001 . 00000000
223. 200. 100. 25 = 11011111 . 11001000 . 01100100 . 00011001
192.168.1.0/24
1st Octet 2nd Octet 3rd Octet 4th Octet
8 bits 8
bit 8
bits 8 bits
272625 24
23 22 21 20 27 26
25 24 23 22 21 20 27 26
25 24 23 22 21 20 27 26
25 24 23 22 21 20
Network Host
Network Address = 192.168.1.0
Subnet
Mask = 255.255.255.0
Usable
IP Addresses = 192.168.1.1 to 192.168.1.254
Broadcast
Address = 192.168.1.255
Tuesday, March 7, 2017
AVG Antivirus
If you are searching free antivirus for your PC, AVG is one of the antivirus software you can get free. There are two types of versions in AVG. One is free version and one is ultimate version.
If you want free version, click here and can download it.
If you want ultimate version, click here and can download it.
Than You.
My Invitation
Download Android Application
If you want free version, click here and can download it.
If you want ultimate version, click here and can download it.
Than You.
My Invitation
Download Android Application
Sunday, January 29, 2017
Microsoft Windows Malicious Software Removal Tool
Hi !
Good evening to all my friends!
Malicious Software or Malware is any software that brings harm to the computer system. It can be in the forms of virus, trojans, rootkits, worms, spyware and adware, etc,. . So you can use Microsoft Windows Malicious Software Removal Tool to remove malware from your computer if you suspect that your computer is infected by malware. You can download Microsoft Windows Malicious Software Removal Tool from Microsoft Website (click here). It is totally free. I hope you will be ok.
Good evening to all my friends!
Malicious Software or Malware is any software that brings harm to the computer system. It can be in the forms of virus, trojans, rootkits, worms, spyware and adware, etc,. . So you can use Microsoft Windows Malicious Software Removal Tool to remove malware from your computer if you suspect that your computer is infected by malware. You can download Microsoft Windows Malicious Software Removal Tool from Microsoft Website (click here). It is totally free. I hope you will be ok.
Friday, January 6, 2017
IT Career
Some of the youths ask me about IT careers. They don't know how to start to enter IT field although they are interested in IT so much. This post is designated for those youths.