Sunday, December 24, 2017
Codes for file upload in PHP (Step 3)
In this example, we will upload file to the designated location. So, we will create a folder to store the files we've uploaded and give name to that folder. I give it as tnw87. Ok, lets start write the following codes and discover how it works.
<?php
$name = $_FILES['file']['name'];
$type = $_FILES['file']['type'];
$size = $_FILES['file']['size'];
$tmp_name = $_FILES['file']['tmp_name'];
if(@isset($name) && @!empty($name)){
$location = 'tnw87/';
if(move_uploaded_file($tmp_name, $location.$name)){
echo $name. ' is successfully uploaded.';
}else{
echo 'Upload Errors';
}
}else{
echo 'Please select file to upload';
}
?>
Wednesday, December 20, 2017
Codes for file upload in PHP (Step 2)
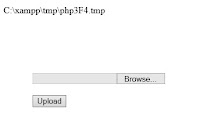
When we upload a file into one location, the file is firstly saved in temporary location as a temp file. Ok, lets discover how it works. We will write the following codes and test it.
<?php
$name = $_FILES['file']['name'];
$type = $_FILES['file']['type'];
$size = $_FILES['file']['size'];
$tmp_name = $_FILES['file']['tmp_name'];
if(@isset($name) && @!empty($name)){
$location = 'tnw87/';
if(move_uploaded_file($tmp_name, $location.$name)){
echo $name. ' is successfully uploaded.';
}else{
echo 'Upload Errors';
}
}else{
echo 'Please select file to upload';
}
?>
<style type = "text/css">
form{margin-top : 100px; margin-left : 50px;}
</style>
<form action = "upload.php" method = "POST" enctype = "multipart/form-data">
<input type = "file" name = "file"></br></br>
<input type = "submit" value = "Upload">
</form>
Friday, December 15, 2017
Codes for File Upload in PHP (Step 1)
This is the first step to write codes for uploading file in php. In this example, you can check name, type and size of files you upload.
<?php
@$name = $_FILES['file']['name'];
@$type = $_FILES['file']['type'];
@$size = $_FILES['file']['size'];
if(@isset($name) && @!empty($name)){
echo 'File name is <strong> '.$name.'</strong>, File type is <strong>'.$type.'</strong> nad File size is <strong> '.$size.'</strong>';
}else{
echo 'Please select file to upload';
}
?>
<?php
@$name = $_FILES['file']['name'];
@$type = $_FILES['file']['type'];
@$size = $_FILES['file']['size'];
if(@isset($name) && @!empty($name)){
echo 'File name is <strong> '.$name.'</strong>, File type is <strong>'.$type.'</strong> nad File size is <strong> '.$size.'</strong>';
}else{
echo 'Please select file to upload';
}
?>
Thursday, December 14, 2017
Configuring Frame Relay in Cisco Devices (Example 2)
#Router 1#
enconfig t
hostname R1
int g0/0
ip address 192.168.1.1 255.255.255.0
no shut
int s0/0/0
description Link From Router1 to Router2
ip address 223.128.1.1 255.255.255.252
encap frame-relay
frame-relay lmi-type cisco
frame-relay interface-dlci 101
no shut
int s0/0/1
encap frame-relay
no shut
exit
int s0/0/1.201 point-to-point
description Link From R1 to R3
ip address 223.128.1.5 255.255.255.252
frame-relay interface-dlci 201
no shut
exit
int s0/0/1.301 point-to-point
description Link From R1 to R4
ip address 223.128.1.9 255.255.255.252
frame-relay interface-dlci 301
no shut
exit
int s0/0/1.401 point-to-point
description Link From R1 to R5
ip address 223.128.1.13 255.255.255.252
frame-relay interface-dlci 401
no shut
exit
int s0/0/1.501 point-to-point
description Link From R1 to R6
ip address 223.128.1.17 255.255.255.252
frame-relay interface-dlci 501
no shut
exit
router rip
version 2
network 192.168.1.0
network 223.128.1.0
network 223.128.1.4
network 223.128.1.8
network 223.128.1.12
network 223.128.1.16
end
copy run start
Wednesday, December 13, 2017
Configuring Frame Relay in Cisco Devices (Example 1)
***Router1***
enconfig t
hostname Router1
int s0/0/0
encap frame-relay
no shut
exit
int s0/0/0.102 point-to-point
description Link From Router1 to Router2
ip address 223.200.100.5 255.255.255.252
frame-relay interface-dlci 102
no shut
exit
int s0/0/0.103 point-to-point
description Link From Router1 to Router3
ip address 223.200.100.9 255.255.255.252
frame-relay interface-dlci 103
no shut
exit
router rip
version 2
network 223.200.100.4
network 223.200.100.8
end
copy run start
Tuesday, December 12, 2017
Using XML with PHP (Example 2)
In this example, you will see using foreach function twice. Ok, let's start write codes. We will write the following xml codes and save it as file name 'example.xml'.
<producer>
<producer>
<food>
<foodname>banana</foodname>
<foodprice>100 kyats</foodprice>
</food>
</producer>
<producer>
<food>
<foodname>lime</foodname>
<foodprice>200 kyats</foodprice>
</food>
</producer>
<producer>
<food>
<foodname>milk</foodname>
<foodprice>300 kyats</foodprice>
</food>
</producer>
</producer>
<producer>
<producer>
<food>
<foodname>banana</foodname>
<foodprice>100 kyats</foodprice>
</food>
</producer>
<producer>
<food>
<foodname>lime</foodname>
<foodprice>200 kyats</foodprice>
</food>
</producer>
<producer>
<food>
<foodname>milk</foodname>
<foodprice>300 kyats</foodprice>
</food>
</producer>
</producer>
Monday, December 11, 2017
Using XML with PHP (Example 1)
We will write the following codes and save it as file name 'example.xml'.
<producer>
<producer>
<name>Thet</name>
<age>30</age>
</producer>
<producer>
<name>Jan</name>
<age>21</age>
</producer>
<producer>
<name>Feb</name>
<age>23</age>
</producer>
<producer>
<name>Mar</name>
<age>25</age>
</producer>
</producer>
<producer>
<producer>
<name>Thet</name>
<age>30</age>
</producer>
<producer>
<name>Jan</name>
<age>21</age>
</producer>
<producer>
<name>Feb</name>
<age>23</age>
</producer>
<producer>
<name>Mar</name>
<age>25</age>
</producer>
</producer>
Friday, December 8, 2017
WordCensoring in PHP
From the following example, we can learn the usage of form, textarea, isset, strtolower and str_replace. Every word 'love' we type in the text box, the codes will change with the word 'hate'.
<?php
$find = 'love';
$replace = 'hate';
if(isset($_POST['user_input']) && !empty($_POST['user_input'])){
$user_input = strtolower($_POST['user_input']);
$user_input_new = str_replace($find, $replace, $user_input);
echo $user_input_new.'</br>';
}else{
echo 'Please fill in the text box!';
}
?>
<?php
$find = 'love';
$replace = 'hate';
if(isset($_POST['user_input']) && !empty($_POST['user_input'])){
$user_input = strtolower($_POST['user_input']);
$user_input_new = str_replace($find, $replace, $user_input);
echo $user_input_new.'</br>';
}else{
echo 'Please fill in the text box!';
}
?>
Thursday, December 7, 2017
Configuring OSPF in Cisco Routers
*** Router 1 ***
Activate the connection to PC1
enconfig t
int g0/0
ip address 192.168.1.1 255.255.255.0
no shut
Activate the connection to Router2
int s0/0/1
ip address 223.200.100.9 255.255.255.252
clock rate 64000
no shut
Activate the connection to Router 3
int s0/0/0
ip address 223.200.100.1 255.255.255.252
no shut
exit
Configure OSPF
router ospf 10
network 192.168.1.0 0.0.0.255 area 0
network 223.200.100.0 0.0.0.3 area 0
network 223.200.100.8 0.0.0.3 area 0
end
Save the configuration
copy run start
Wednesday, December 6, 2017
String Trim in PHP
<?php
$string = 'I am Thet Naing Win.';
$string_trimmed = rtrim($string);
echo $string_trimmed;
?>
$string = 'I am Thet Naing Win.';
$string_trimmed = rtrim($string);
echo $string_trimmed;
?>
Tuesday, December 5, 2017
Configuring PPP in Cisco Routers
This is the configuration of PPP (PAP) in Cisco Routers
*** Mandalay Router ***
enconfig t
username Mandalay password Mandalay
hostname Mandalay
int s0/0/0
ip address 223.200.100.1 255.255.255.252
no shut
encap ppp
ppp authentication pap
ppp pap sent-username Naypyidaw password Naypyidaw
exit
int s0/0/1
ip address 223.200.100.9 255.255.255.252
no shut
encap ppp
ppp authentication pap
ppp pap sent-username Yangon password Yangon
exit
router rip
version 2
network 223.200.100.0
end
copy run start
Monday, December 4, 2017
String Word Count in PHP
<?php
$string = 'I am Thet Naing Win and who are you?';
$string_word_count = str_word_count($string, 1);
print_r($string_word_count);
?>
$string = 'I am Thet Naing Win and who are you?';
$string_word_count = str_word_count($string, 1);
print_r($string_word_count);
?>
Sunday, December 3, 2017
Writing Basic XML Codes
*** Example 1 ***
<producer>
<producer>
<name>Thet</name>
<age>30</age>
</producer>
<producer>
<name>Naing</name>
<age>32</age>
</producer>
<producer>
<name>Win</name>
<age>33</age>
</producer>
</producer>
<producer>
<producer>
<name>Thet</name>
<age>30</age>
</producer>
<producer>
<name>Naing</name>
<age>32</age>
</producer>
<producer>
<name>Win</name>
<age>33</age>
</producer>
</producer>
Saturday, December 2, 2017
Virtualization (Installing Ubuntu in VirtualBox)
Virtualization
Virtualizatin is installing two or more operating systems in one physical machine. The main effects of using virtualizaton are security and cost saving. Oracle VirtualBox is one of virtualization softwares. It is open source software and totally free. Please click here to download. Install it after downloading.Ubuntu
Ubuntu is one of open source operating systems. Please click here to download ubuntu ISO file.Installing Ubuntu in VirtualBox
1. Start VirtualBox
- Open VirtualBox and Click New.
2.Choose Name and Operating System
- Type name in the Name box (for example, T N W)
- Choose operating system in Type box (here we will choose Linux)
- Chose version in Version box (here I will choose Ubuntu-64 bit)
- And then click Next.
Friday, December 1, 2017
String Replace in PHP
<?php
$string = 'I am thet naing win';
$find = array('thet', 'naing', 'win');
$replace = array('THET', 'NAING', 'WIN');
$string_new = str_replace($find, $replace, $string);
echo $string_new;
?>